🛠️ Linux Shell Scripting – Automate Admin Tasks Like a Pro
Introduction
Linux Shell Scripting: System administrators spend a lot of time performing repetitive tasks like backups, user management, and system monitoring. Shell scripting can automate these tasks, saving time and reducing errors.
In this guide, we’ll cover:
✅ Writing shell scripts for automation
✅ Automating backups and user management
✅ Monitoring system performance with scripts
✅ Scheduling scripts with cron jobs
By the end, you’ll be able to write efficient shell scripts for system administration.
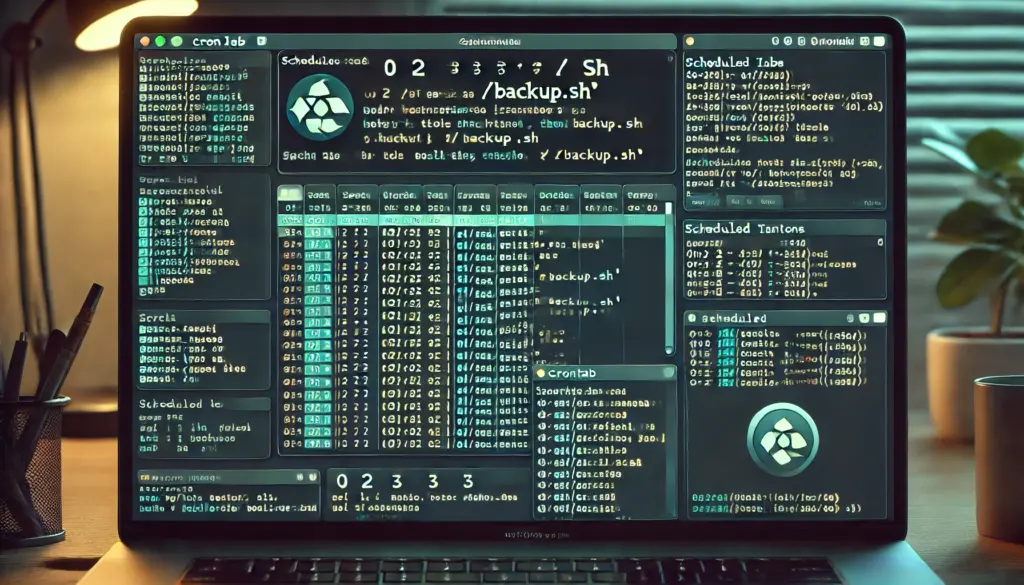
📌 1. Automating Backups with Shell Scripts
Backing up files and directories is essential for system recovery.
Example: Simple Backup Script
#!/bin/bash
backup_dir="/backup"
source_dir="/home/user"
timestamp=$(date +%Y-%m-%d_%H-%M-%S)
backup_file="$backup_dir/home_backup_$timestamp.tar.gz"
echo "Starting backup..."
tar -czf $backup_file $source_dir
echo "Backup completed: $backup_file"
Steps to Automate This Script:
1️⃣ Save this script as backup.sh
.
2️⃣ Make it executable:
chmod +x backup.sh
3️⃣ Run it manually:
./backup.sh
4️⃣ Schedule it with cron to run daily at 2 AM:
crontab -e
Add this line:
0 2 * * * /path/to/backup.sh
✅ Now, your home directory will be backed up automatically every night!
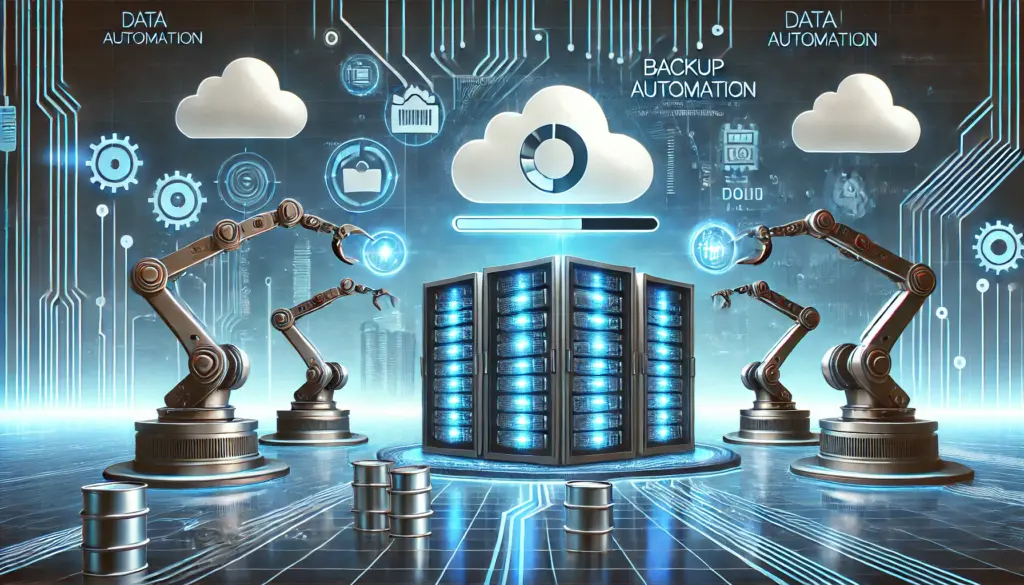
📌 2. Automating User Management Tasks
Creating users manually can be time-consuming. Let’s automate it!
Example: Create Multiple Users from a File
#!/bin/bash
user_list="users.txt"
while IFS= read -r user
do
sudo useradd -m "$user"
echo "User $user created successfully!"
done < "$user_list"
Steps to Use This Script:
1️⃣ Create a file users.txt
and add usernames, one per line.
2️⃣ Save the script as create_users.sh
.
3️⃣ Make it executable:
chmod +x create_users.sh
4️⃣ Run the script:
./create_users.sh
✅ Now, all users in users.txt
will be created automatically!
📌 3. Automating System Monitoring
Monitor CPU, memory, and disk usage with a shell script.
Example: System Health Monitoring Script
#!/bin/bash
echo "System Health Report - $(date)"
echo "----------------------------------"
echo "Uptime:"
uptime
echo ""
echo "CPU Usage:"
top -b -n1 | grep "Cpu(s)"
echo ""
echo "Memory Usage:"
free -h
echo ""
echo "Disk Usage:"
df -h
Steps to Use This Script:
1️⃣ Save it as system_monitor.sh
.
2️⃣ Make it executable:
chmod +x system_monitor.sh
3️⃣ Run it manually:
./system_monitor.sh
4️⃣ Automate it to run every hour using cron:
crontab -e
Add this line:
0 * * * * /path/to/system_monitor.sh >> /var/log/system_health.log
✅ Now, the system will be monitored every hour, and logs will be saved!
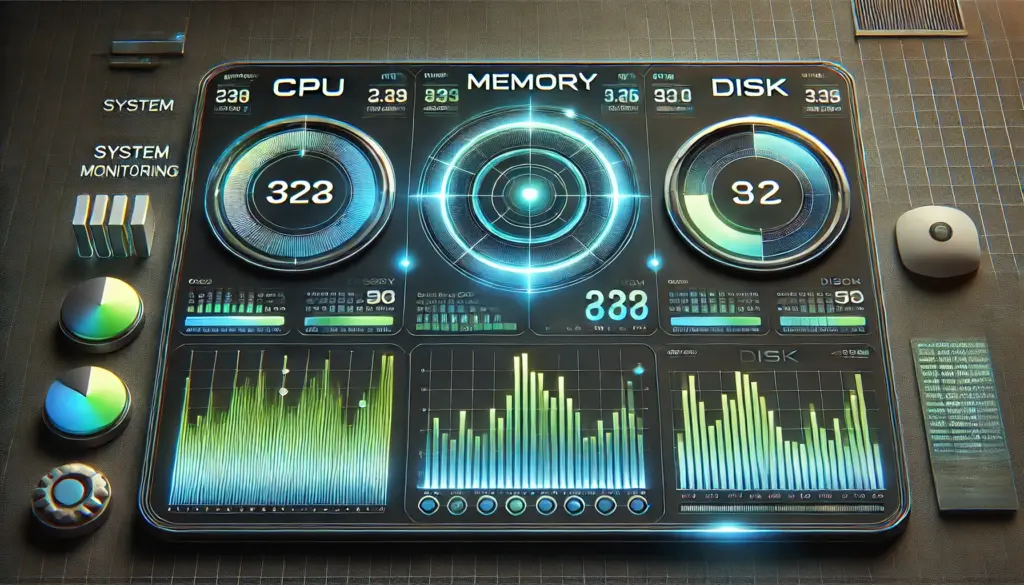
📌 4. Automating Log Cleanup
Log files can grow large and consume disk space. Let’s automate log cleanup.
Example: Delete Old Log Files
#!/bin/bash
log_dir="/var/log"
days=30
find $log_dir -type f -name "*.log" -mtime +$days -exec rm -f {} \;
echo "Old log files deleted!"
Steps to Use This Script:
1️⃣ Save it as log_cleanup.sh
.
2️⃣ Make it executable:
chmod +x log_cleanup.sh
3️⃣ Run it manually:
./log_cleanup.sh
4️⃣ Automate it to run weekly:
crontab -e
Add this line:
0 0 * * 0 /path/to/log_cleanup.sh
✅ Now, old log files will be deleted every Sunday at midnight!
📌 5. Sending System Alerts via Email
If system load gets too high, send an email alert.
Example: Email Alert for High CPU Usage
#!/bin/bash
threshold=80
cpu_load=$(top -b -n1 | grep "Cpu(s)" | awk '{print $2 + $4}')
if (( $(echo "$cpu_load > $threshold" | bc -l) )); then
echo "High CPU Usage Alert! Current Load: $cpu_load%" | mail -s "CPU Alert" admin@example.com
fi
Steps to Use This Script:
1️⃣ Install mail utilities:
sudo apt install mailutils
2️⃣ Save it as cpu_alert.sh
.
3️⃣ Make it executable:
chmod +x cpu_alert.sh
4️⃣ Automate it to check every 5 minutes:
crontab -e
Add this line:
*/5 * * * * /path/to/cpu_alert.sh
✅ Now, you’ll receive an email alert if CPU usage goes beyond 80%!
🎯 Final Thoughts
With shell scripting, you can automate backups, user management, system monitoring, and more, making your job as an administrator much easier.
Learn More:
Common Challenges in Incident Management
Essential Technical Skills for Aspiring Incident Managers
Understanding the ITIL Framework for Incident Management
Key Roles and Responsibilities in Incident Management
📌 Call to Action (CTA)
💬 Which admin task do you want to automate next? Let us know in the comments!
🔔 Follow TechNops.com for more Linux tutorials!
Informative
Thanks