🚀 Advanced Bash Scripting – Functions, Arguments, and Debugging
Introduction
Now that you’re familiar with basic Bash scripting, it’s time to take things up a notch with Advanced Bash Scripting
In this guide, we’ll cover:
✅ Using functions for better code organization
✅ Passing command-line arguments to scripts
✅ Debugging techniques to fix errors
✅ Best practices for writing clean scripts
By the end, you’ll be able to write modular, efficient, and error-free Bash scripts.
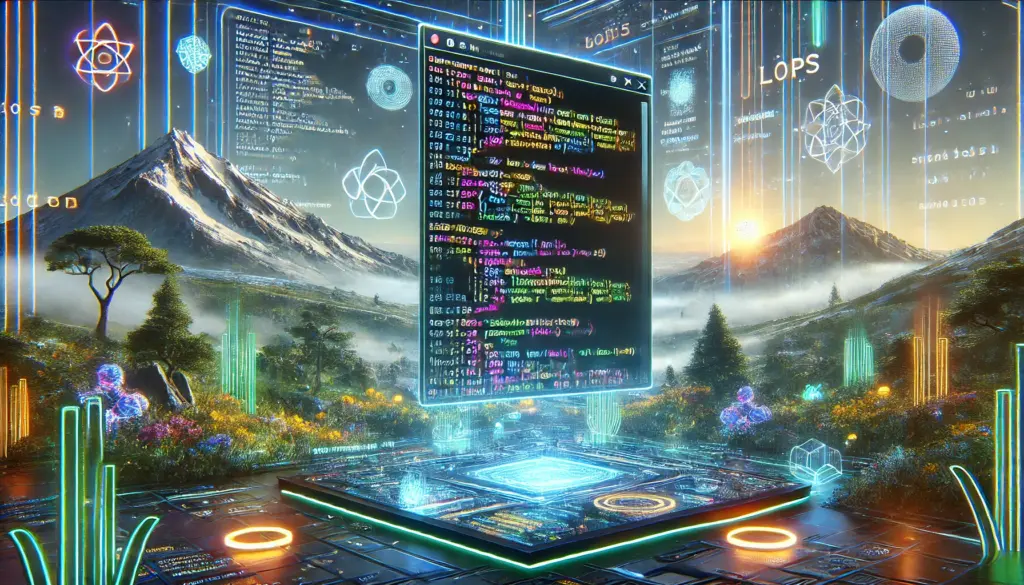
📌 1. Functions in Bash
Functions help organize code by grouping commands into reusable blocks.
Syntax of a Bash Function
function function_name() {
# Code block
}
OR
function_name() {
# Code block
}
Example: Function to Greet Users
#!/bin/bash
greet_user() {
echo "Hello, $1! Welcome to Bash scripting."
}
greet_user "Alice"
✅ Output:
Hello, Alice! Welcome to Bash scripting.
📌 $1
is the first argument passed to the function.
📌 2. Using Command-Line Arguments in Scripts
Scripts can accept user input via command-line arguments.
Example: Passing Arguments to a Script
#!/bin/bash
echo "Script Name: $0"
echo "First Argument: $1"
echo "Second Argument: $2"
Run the script with arguments:
./script.sh John 25
✅ Output:
Script Name: ./script.sh
First Argument: John
Second Argument: 25
📌 $0
→ Script name
📌 $1
, $2
, $3
→ First, second, third arguments
Handling Multiple Arguments with $@
and $#
#!/bin/bash
echo "All Arguments: $@"
echo "Total Arguments: $#"
✅ $@
→ All arguments
✅ $#
→ Number of arguments
📌 3. Conditional Statements in Bash
Use if
, elif
, and else
to handle logic.
Example: Checking If a File Exists
#!/bin/bash
if [ -f "$1" ]; then
echo "File $1 exists."
else
echo "File $1 does not exist."
fi
Run the script:
./check_file.sh myfile.txt
📌 4. Loops in Advanced Bash Scripting
For Loop with File Processing
#!/bin/bash
for file in *.txt
do
echo "Processing $file..."
done
While Loop with User Input
#!/bin/bash
while true
do
echo "Enter a number (or type 'exit' to quit):"
read input
if [ "$input" == "exit" ]; then
break
fi
echo "You entered: $input"
done
📌 5. Debugging Bash Scripts
Even experienced scripters encounter bugs! Here are ways to debug your script:
1️⃣ Enable Debug Mode (-x
flag)
Run your script in debug mode to see every command executed:
bash -x script.sh
2️⃣ Add Debugging Statements (set -x
and set +x
)
Wrap parts of your script with set -x
and set +x
to debug specific sections.
#!/bin/bash
echo "Before debug mode"
set -x # Start debugging
ls /invalid/path
set +x # Stop debugging
echo "After debug mode"
3️⃣ Use the trap
Command to Catch Errors
#!/bin/bash
trap 'echo "An error occurred on line $LINENO"; exit 1' ERR
ls /invalid/path
✅ Now, the script will display the line number where the error occurred!
📌 6. Best Practices for Writing Bash Scripts
✔ Use Comments (#
): Explain your code for future reference.
✔ Use Meaningful Variable Names: Avoid generic names like var1
, var2
.
✔ Check for Errors: Use [ -f ]
for files, [ -d ]
for directories.
✔ Make Scripts Executable: Use chmod +x script.sh
.
✔ Format Output Clearly: Use echo -e
for colored output.
Example:
echo -e "\e[32mSuccess!\e[0m" # Prints green text
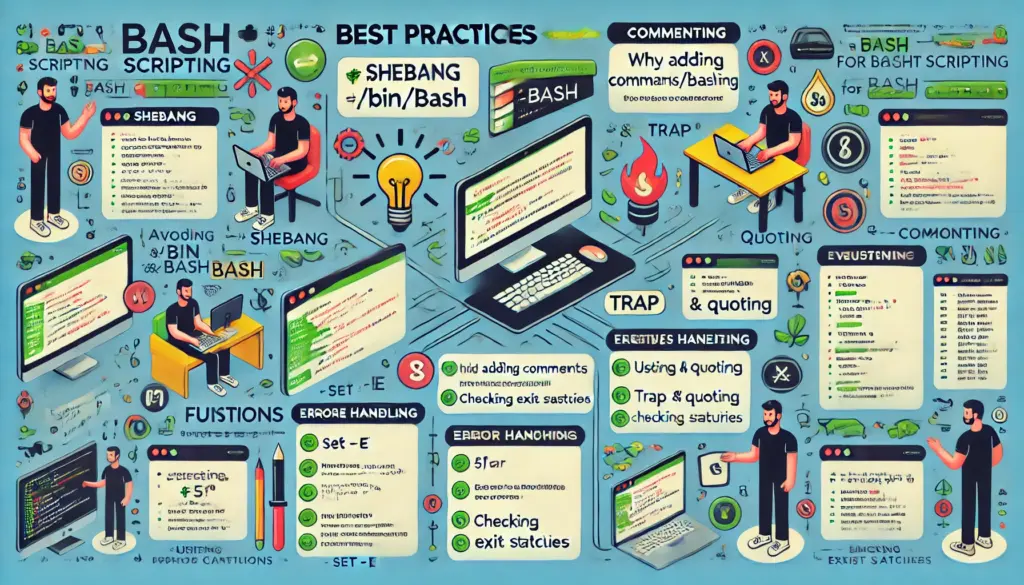
🎯 Final Thoughts
Now you know how to write functions, pass arguments, debug scripts, and follow best practices.
💡 Next Blog: Linux Shell Scripting – Automate Admin Tasks Like a Pro
Learn More:
Common Challenges in Incident Management
Essential Technical Skills for Aspiring Incident Managers
Understanding the ITIL Framework for Incident Management
Key Roles and Responsibilities in Incident Management
📌 Call to Action (CTA)
💬 What’s the most useful Bash function you’ve written? Share in the comments!
🔔 Follow TechNops.com for more Linux tutorials!