🤖 Bash Scripting for Beginners – Automate Your Tasks
Introduction
Are you tired of repeating the same commands in Linux? Bash scripting allows you to automate tasks, making your work faster and more efficient.
In this guide, we’ll cover:
✅ What is Bash scripting?
✅ Writing your first script
✅ Variables, loops, and conditionals
✅ Making your script executable
By the end, you’ll be able to write basic Bash scripts and automate daily tasks.
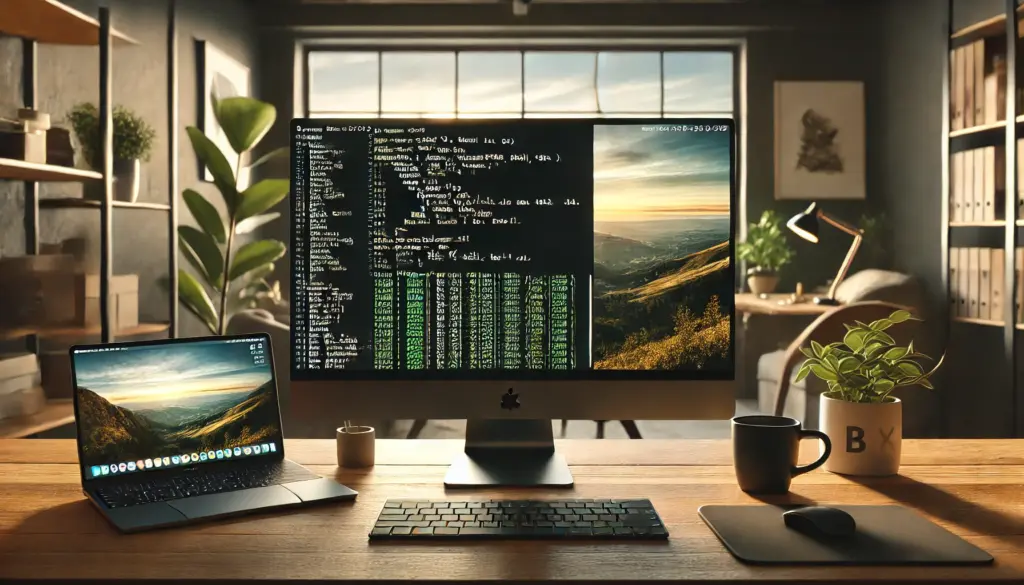
📌 What is Bash Scripting?
Bash (Bourne Again SHell) is the default command-line interpreter in most Linux distributions. A Bash script is a file containing commands that can be executed in sequence.
📌 Why use Bash scripting?
✔ Automate repetitive tasks
✔ Schedule jobs with cron
✔ Manage system processes
✔ Customize command-line workflows
📌 Writing Your First Bash Script
Let’s create a simple script that prints a message.
Step 1: Create a new script file
nano myscript.sh
Step 2: Add the following code
#!/bin/bash
echo "Hello, welcome to Bash scripting!"
📌 The #!/bin/bash
line is called a shebang – it tells the system to use Bash to interpret the script.
Step 3: Save and exit nano
Press CTRL + X
, then Y
, and hit Enter
.
Step 4: Make the script executable
chmod +x myscript.sh
Step 5: Run the script
./myscript.sh
✅ Output:
Hello, welcome to Bash scripting!
📌 Using Variables in Bash
Variables store values that can be reused in scripts.
Example: Using Variables
#!/bin/bash
name="John"
echo "Hello, $name!"
✅ Output:
Hello, John!
📌 Best Practices for Variables:
✔ Use meaningful names: user_name
, file_path
✔ Use $VAR
to access the value
📌 Using Conditional Statements
Conditionals allow scripts to make decisions.
Example: Checking if a File Exists
#!/bin/bash
if [ -f "myfile.txt" ]; then
echo "File exists."
else
echo "File does not exist."
fi
✅ Output (if file exists):
File exists.
✅ Output (if file does not exist):
File does not exist.
📌 Common Conditional Checks:
[ -f file ]
→ File exists[ -d directory ]
→ Directory exists[ -z "$var" ]
→ Variable is empty
📌 Using Loops in Bash
Loops repeat commands multiple times.
Example: For Loop
#!/bin/bash
for i in {1..5}
do
echo "Iteration $i"
done
✅ Output:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
Example: While Loop
#!/bin/bash
count=1
while [ $count -le 3 ]
do
echo "Loop count: $count"
((count++))
done
📌 Accepting User Input in Scripts
Bash scripts can interact with users using the read
command.
Example: Simple User Input Script
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name!"
✅ Output:
Enter your name:
John
Hello, John!
📌 Running a Script Automatically with Cron Jobs
Want to run your script at a specific time? Use cron jobs!
Step 1: Open the cron editor
crontab -e
Step 2: Add a new cron job
Run backup.sh
every day at 2 AM:
0 2 * * * /home/user/backup.sh
✅ Now, the script runs automatically every day at 2 AM!
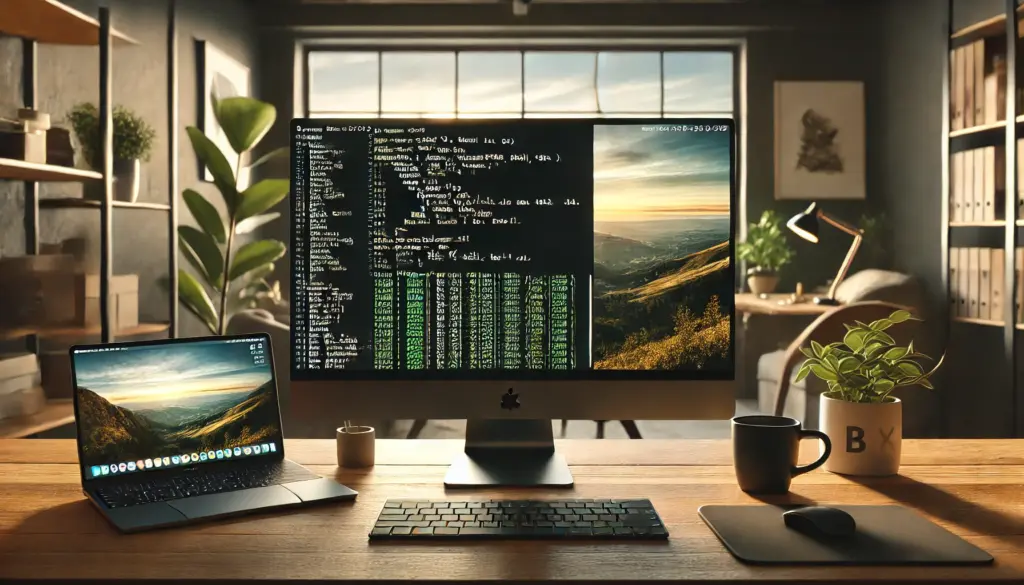
🎯 Final Thoughts
Bash scripting is a powerful way to automate tasks, save time, and manage systems efficiently.
💡 Next Blog: Advanced Bash Scripting – Functions, Arguments, and Debugging
Learn More:
Common Challenges in Incident Management
Essential Technical Skills for Aspiring Incident Managers
Understanding the ITIL Framework for Incident Management
Key Roles and Responsibilities in Incident Management
📌 Call to Action (CTA)
💬 Have you written a Bash script before? Share your experience in the comments!
🔔 Follow TechNops.com for more Linux tutorials!